In parts 4 and 5 of this series, we’ll continue to build out some major backend components of our Quiz app. In Part 3, we created a simple React.js component but it currently attempts to post to grades to an invalid URL. This is because our approach to the project is to build the react (js/css) static files, but first we will need to update our Flask template to take care of this.
Create the Flask template
Back in Part 1, we set up our Flask app to look for template and static files in ./frontend/templates and ./frontend/static, respectively.
In Part 2, we then created an LTI Launch route to do the following:
render_template('index.html', **tpl_kwargs)
We’re expected to return a template. I chose index.html for the name, but feel free to use a naming convention that works for your project. We also pass a number of arguments to the rendered page. We’re currently only using the highlighted properties (below) in our project. The others are examples of keys that could come in handy if you want to display the user’s name or confirm a deep link launch. We’ll cover this in a separate, future post.
'page_title': 'LTI 1.3 Flask-React Quiz',
'is_deep_link_launch': message_launch.is_deep_link_launch(),
'launch_data': message_launch.get_launch_data(),
'launch_id': message_launch.get_launch_id(),
'curr_user_name': message_launch_data.get('name', ''),
Back in the Flask backend of our project, my layout looks like this. Yours may vary.
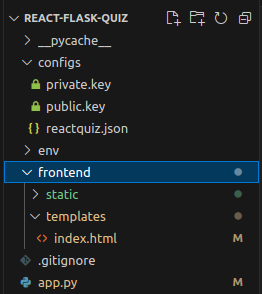
You will want to create a frontend directory within your root project directory. While this isn’t necessary to structure it this way (creating static and template directories in your Flask project root is fine), I like to denote that the frontend is in fact a separate react project, not your typical Flask project layout.
In your frontend directory, create static and templates directories at the same branch in your directory tree.
Create your empty template file in the templates directory. In my case, index.html. Enter or paste the following html:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>{{ page_title }}</title>
<script>
var launchId = "{{ launch_id }}";
</script>
<link
href="{{ url_for('static', filename='css/<Put Your React Static CSS File Here>) }}"
rel="stylesheet"
/>
</head>
<body>
<div id="root"></div>
<script
type="text/javascript"
src="{{ url_for('static', filename='js/<Put Your React Static JS File Here') }}"
charset="utf-8"
></script>
</body>
</html>
This simple template does the following:
- Grabs page_title and launch_id from the kwargs passed to the template when rendered.
- References the static react CSS/JS files that we have yet to create.
- The body of our Flask template loads a div with “root”. Always make sure the order is following this convention, at the top of the body of your HTML template / document, prior to loading the javascript <script>.
Build and Implement the React.js frontend
Next, you’ll want to build your React project (as you would for production) using the proper command at your terminal, the root of your frontend/react project, wherever you chose to create that. In my case, I’m using a separate project altogether. Another approach would’ve been to build everything in the frontend directory within your backend/Flask project. I will cover a more “elegant” (questionable) method by using webpack, in a later post. I’m also open to suggestions for better tooling of this method. I aspire to be a solid Vue.js and Django developer, so some of this is outside of my … area of concentration, perhaps? Anywho:
npm run build
Finally copy your react project’s static directory (including all sub-directories) over to the Flask project, so that the structure is similar to this:
React.js project: /build/static.. to Flask project: /frontend/static
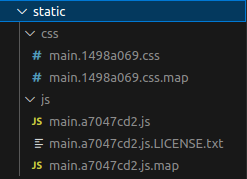
The file names that are generated are unique to your project, so they’ll be different from mine in the image above.
Finally, update the index.html template with names that match your main.xxxxx.js and main.xxxxx.css files. I’ve highlighted the 2 lines of code that need to be changed in the original screenshot of the template above.
In Part 5, we’ll make one last update to our React component, so that we’re dynamically retrieving data via API and a database. But first, we’ll create a default questions python module, API routes and method handlers, and some backend database handlers.